Code Styleguide
The SAIT website is built using Bootstrap 5, Typescript and Webpack. Although most of what you will need to build out a page is made possible through pre-made blocks, you might want to familiarize yourself with the grid system as well as some helper classes that can help with basic spacing and styling within a WYSIWYG.
Before you read
The code of the website is maintained by the Digital Experience team in Marketing. If you have any questions about the site's code or buildout, please reach out to site.feedback@sait.ca.
Email usHTML & SASS/CSS best practices
HTML
- Do not use more than one
<h1>
tag per page.<h1>
tags are typically reserved for the hero banner and are not used in the body of the page. - Make sure
<h1>
to<h6>
tags are in sequential order, do not skip over one - Use semantic elements where possible. Examples:
- Use
<header>
and<footer>
instead of <div> - Use
<strong>
and<em>
instead of <b> and <i>
- Use
- Do not use
<div>
where a semantic element can be used instead - Avoid using block level elements (
<p>
,<div>
, etc) inside inline elements (<span>
,<em>
,<a>
) - Use
<aria>
tags appropriately and if you are unsure about the usage avoid using it as improperly used, it can make for a negative experience - Use descriptive alt tags for images. If image is used for decorative purposes, add empty alt tag (alt="")
SASS/CSS
- Try to use variables (in _variables.scss) when possible
- Follow WCAG 2 AA guidelines in terms of colour contrast, font size, etc.
- Use meaningful class and ID names
- Try to avoid using overly specific CSS selectors and opt for flexibility
- Minimize nesting as much as possible (only go ~4 levels deep)
- Avoid using !important; property, instead try to follow CSS specificity rules
- Before creating a new selector, see if something already exists within the codebase
- Style with mobile-first in mind - use min-width media queries over max-width
- Add fallbacks when necessary
Breakpoints
Breakpoints are widths that determine how your responsive layout behaves across device sizes. The code infixes will come in handy with column and helper classes.
Breakpoint | Class infix | Dimensions |
---|---|---|
X-Small | None | <576px |
Small | sm |
≥576px |
Medium | md |
≥768px |
Large | lg |
≥992px |
Extra large | xl |
≥1200px |
Extra extra large | xxl |
≥1400px |
Extra extra extra large | xxxl |
≥1640px |
Grid system and columns
Bootstrap's grid system uses a series of containers, rows and columns to layout and align content. A row is broken up into 12 columns which allows you to build layouts of all shapes and sizes. Columns must be wrapped in rows, as rows add proper padding that controls space between columns. Below are some examples of useage. For a more in-depth look at Bootstrap's grid system check out their docs.
<div
class="container">
<div
class="row">
<div
class="col">
Column
</div>
<div
class="col">
Column
</div>
<div
class="col">
Column
</div>
</div>
</div>
For responsive behaviour, add breakpoint infixes (from above) to the col
classes. When adding a breakpoint, it will affect that breakpoint and all those above it. For example, if you want a column that is full width in small screens, then is half width in medium screens, you'd give it the class col-md-6
.
<div
class="container">
<div
class="row">
<div
class="col-5">
col-5
</div>
<div
class="col-4">
col-4
</div>
</div>
<div
class="row">
<div
class="col-md-6">
col-md-6
</div>
<div
class="col-md-3">
col-md-3
</div>
<div
class="col-md-3">
col-md-3
</div>
</div>
</div>
Alignment
Use flexbox alignment utilities to vertically and horizontally align columns. To learn more about how flexbox works, check out this CSS Tricks flexbox guide.
Vertical alignment
For vertical alignment, add the class align-items-start
, align-items-center
or align-items-end
to the parent row
.
<div
class="container">
<div
class="row align-items-start">
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
</div>
<div
class="row align-items-center">
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
</div>
<div
class="row align-items-end">
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
<div
class="col">
One of three columns
</div>
</div>
</div>
Horizontal alignment
To align columns horizontally, add the class justify-content-start
, justify-content-center
, justify-content-end
, justify-content-around
, justify-content-between
or justify-content-evenly
to the parent row
.
<div
class="container">
<div
class="row justify-content-start">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
<div
class="row justify-content-center">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
<div
class="row justify-content-end">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
<div
class="row justify-content-around">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
<div
class="row justify-content-between">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
<div
class="row justify-content-evenly">
<div
class="col-4">
One of two columns
</div>
<div
class="col-4">
One of two columns
</div>
</div>
</div>
Spacing
Bootstrap is built in with responsive-friendly margin
and padding
helper classes to adjust spacing between elements.
The classes are named using the format {property}{sides}-{breakpoint}{size}
(omitting the breakpoint
for xs sizes).
Property is one of m
for margin and p
for padding. Margin is the space around an element's border, while padding is the space between an element's content and border.
Sides refers to (leave this blank to affect all sides):
t
: topb
: bottoms
: start (left side)e
: end (right side)x
: both left and right sidey
: both top and bottom
Size ranges from 0 - 5
as well as auto
.
Below are some examples of the classes being used:
<div
class="container">
<div
class="row">
<div
class="col mt-4 ms-3">
col mt-4 ms-3
</div>
<div
class="col my-3">
col my-3
</div>
</div>
<div
class="row">
<div
class="col p-md-5">
col p-md-5
</div>
</div>
<div
class="row">
<div
class="col-6 me-2">
col-6 me-2
</div>
<div
class="col-4">
col-4
</div>
</div>
</div>
Helper Classes
Class | Description | Example |
---|---|---|
.color-dark-red |
#A6192E text colour. |
Lorem ipsum dolor sit. |
.color-light-red |
#DA291C text colour. |
Lorem ipsum dolor sit. |
.color-light-blue |
#00A3E text colour. |
Lorem ipsum dolor sit. |
.text-white |
White text colour. |
Lorem ipsum dolor sit. |
.highlight |
Highlighted block of text. |
Lorem ipsum dolor sit. |
.lead |
Lead paragraph text. |
Lorem ipsum dolor sit. |
.text-uppercase |
Uppercase text. |
Lorem ipsum dolor sit. |
.text-start |
Left-aligned text. Can also use responsive classes (ex. text-md-start ). |
Lorem ipsum dolor sit. |
.text-center |
Center text. Can also use responsive classes (ex. text-lg-center ). |
Lorem ipsum dolor sit. |
.text-end |
Right-aligned text. Can also use responsive classes (ex. text-sm-end ). |
Lorem ipsum dolor sit. |
.bg-warm-grey |
#F7F7F8 background colour. |
|
.bg-cool-grey |
#F6F7F9 background colour. |
|
.bg-dark |
#212529 background colour. |
|
.bg-white |
#FFFFFF background colour. |
|
.bg-dark-red |
#A6192E background colour. |
|
.bg-light-red |
#DA291C background colour. |
|
.bg-purple |
#6D2077 background colour. |
|
.bg-dark-blue |
#005EB8 background colour. |
|
.bg-light-blue |
#00A3E background colour. |
|
.bg-tiled |
Tiled background image. | |
.bg-cover |
Cover background image. | |
.bg-center |
Centered background image. | |
.bg-left-center |
Left positioned background image. | |
.bg-right-center |
Right positioned background image. | |
.bg-fixed |
Fixed background image. Works best on full-width sections. |
Code examples current as of Sept 2024
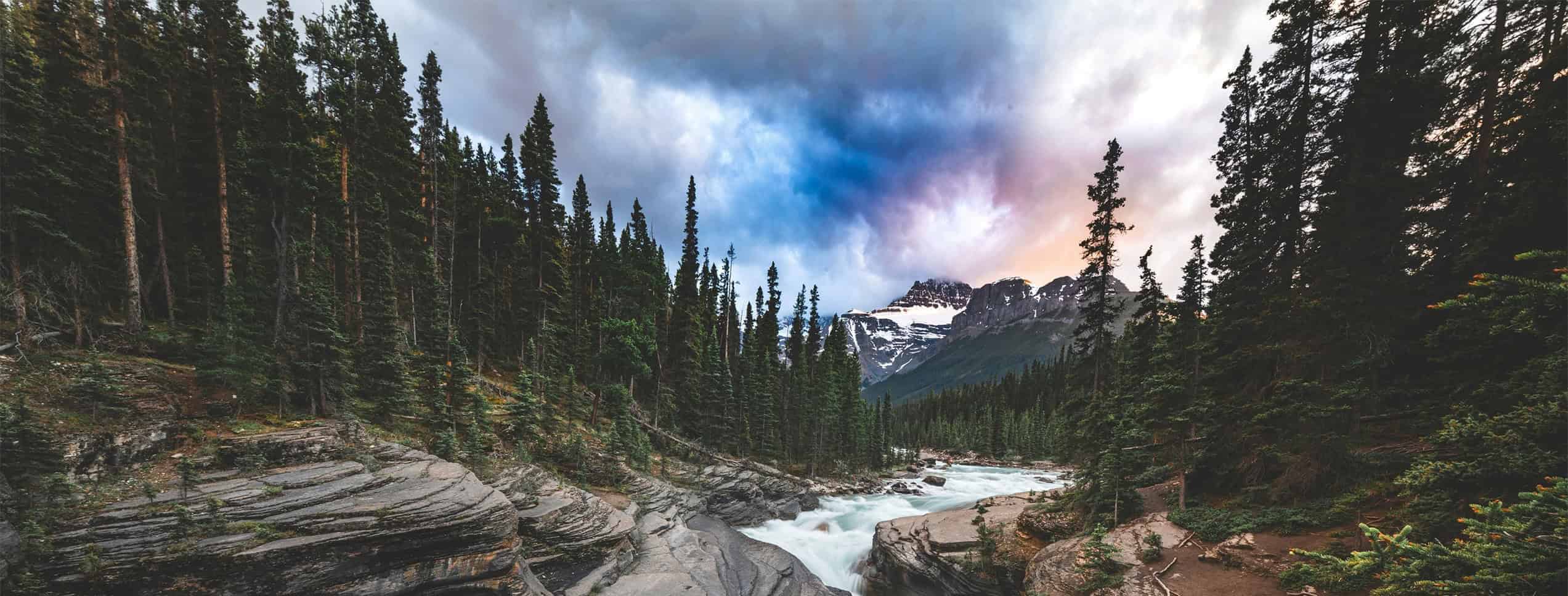
Oki, Âba wathtech, Danit'ada, Tawnshi, Hello.
SAIT is located on the traditional territories of the Niitsitapi (Blackfoot) and the people of Treaty 7 which includes the Siksika, the Piikani, the Kainai, the Tsuut’ina and the Îyârhe Nakoda of Bearspaw, Chiniki and Goodstoney.
We are situated in an area the Blackfoot tribes traditionally called Moh’kinsstis, where the Bow River meets the Elbow River. We now call it the city of Calgary, which is also home to the Métis Nation of Alberta.